Hi friends.. Today i am going to tell you about how to insert data available in HTML from using PHP in to MySQL database. Here i will use post method for transfer data using HTML form to my PHP script. Lets see here how to create form without having table. I mean you can create a HTML form and you want to align it in proper manner. In here you can see inside my css code i have aligned it without using table .OK here is my code for create HTML form
Below you can seemy css code for displaying the proper out put like this
<!-- To change this template, choose Tools | Templates and open the template in the editor. --> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <html> <head> <style type="text/css"> body {background-color:black;} #main-body {width: 350px; height: 250px; background-color: cornflowerblue; border: 6px brown dashed; margin-left: 300px; margin-top: 200px; padding: 10px; } #main-body-inner { width: 340px; height: 240px; background-color: white; margin-top:5px; margin-left: 5px; } #myform {padding: 30px;} #myform label,input{ display: block; width: 120px; float: left; margin-bottom: 10px; } #myform label{ text-align: right; padding-right: 20px; } br { clear: left; } #submit {width: 75px; float: left; margin-left: 175px;} </style> <title>INSERT DATA USING PHP TO MYSQL DATABASE</title> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> </head> <body> <div id="main-body"> <div id="main-body-inner"> <form id="myform" name="myform" action="insert.php" method="post"> <label for="first-name">First Name</label> <input type="text" id="fname" name="fname" /> <br /> <label for="last-name">Last Name</label> <input type="text" id="lname" name="lname" /><br /> <label for="age">Age</label> <input type="text" id="age" name="age" /><br /> <input type="submit" id="submit" value="Submit" /> </form> </div> </div> </body> </html>
Now we have created our html form and we have styled it. Below you can see my PHP code.
<?php /* * author :damith.uwu@gmail.com * * * */ include 'connection.php'; if(isset ($_POST['fname']) && isset ($_POST[ 'lname']) && isset ($_POST[ 'age'])) // getting the values from the post { $connection = new createConnection(); // creating connection $connect = $connection->connectToDatabase(); // connect to database $connection->selectDatabase(); // select database $fname = mysql_real_escape_string($_POST['fname']); // escape sql injection $lname =mysql_real_escape_string($_POST['lname']); $age = mysql_real_escape_string($_POST['age']); $query="insert into test values ('$fname','$lname','$age')"; // query for insert data mysql_query($query); // execute the insert query echo ("User registration Successfull.."); echo "<a href='index.html'><h1>Go Back </h1> </a>"; // link for go back $connection->closeConnection(); // closing the connection } else { echo "Error occured!"; // display error message } ?>
In here i have used a connection class. Below you can see my connection class.
<?php /** * @author damith * @copyright 2011 */ class createConnection { var $host="localhost"; var $username="username here"; Var $password=" password here"; var $database="databse name"; var $myconn; function connectToDatabase() { $conn= mysql_connect($this->host,$this->username,$this->password); if(!$conn) { die ("Cannot connect to the database"); } else { $this->myconn = $conn; } return $this->myconn; } function selectDatabase() { mysql_select_db($this->database); if(mysql_error()) { echo "Cannot find the database ".$this->database; } } function closeConnection() { mysql_close($this->myconn); } } ?>
When you completed the insert process you can see the message like this.
OK you done.. Download the full source from here
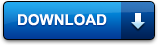